OBJECT ORIENTED PROGRAMMING (CST-004) UTU
Object-Oriented Programming (OOP) is a programming paradigm that organizes software design around objects, encapsulating data and behavior.
- Intermediate
- 9
- September 15, 2024
- Certificate of completion
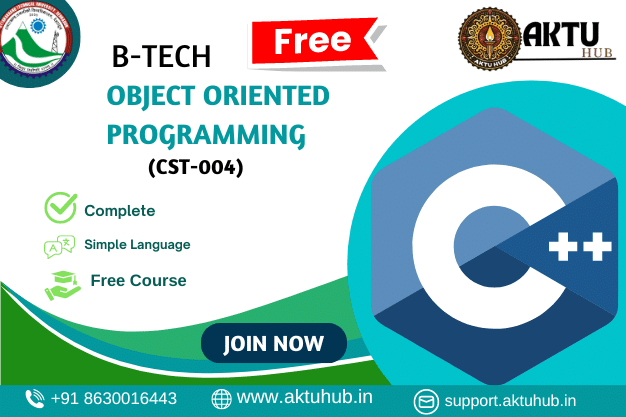
About Course
COURSE OBJECTIVES: The objectives of this course are to:
- Provide flexible and powerful abstraction.
- Allow programmers to think the problem in terms of the structure rather than in terms of structure of the computer.
- Decompose the problem into a set of objects.
- Objects interact with each other to solve the problem.
- Create new type of objects to model elements from the problem space
RSE OUTCOMES: On successful completion of the course, the student will be able to:
- Recognize features of object-oriented design such as encapsulation, polymorphism, inheritance, and composition of systems based on object identity.
- Apply some common object-oriented design patterns.
- Specify simple abstract data types and design implementations using abstraction functions to document them.
- Design a convenient way for the handling problems using templates and use simple try-catch blocks for Exception Handling.
- Manage I/O streams and File I/O oriented interactions.
Course Content :-
Unit 1- Object Oriented Programming Concepts:
Classes and Objects, Methods and Messages, Abstraction and Encapsulation, Inheritance, Abstract Classes, Polymorphism. Introduction to C++: Classes and Objects, Structures and Classes, Unions and Classes, Friend Functions, Friend Classes, Inline Functions, Static Class Members, Scope Resolution Operator, Nested Classes, Local Classes, Passing Objects to Functions, Returning objects, object assignment. Arrays, Pointers, References, and the Dynamic Allocation Operators: Arrays of Objects, Pointers to Objects, Type Checking, this Pointer, Pointers to Derived Types, Pointers to Class Members, References, Dynamic Allocation Operators.
Unit 2- Function Overloading and Constructors:
Function Overloading, Constructors, parameterized constructors, Copy Constructors, Overloading Constructors, Finding the Address of an Overloaded Function, Default Function Arguments, Function Overloading and Ambiguity. Operator overloading: Creating member Operator Function, Operator Overloading Using Friend Function, Overloading New and Delete, Overloading Special Operators, Overloading Comma Operator.
Unit 3- Inheritance and Polymorphism: Inheritance:
Base-Class Access Control, Inheritance and Protected Members, Inheriting Muitiple Base Classes, Constructors, Destructors and Inheritance, Granting Access, Virtual Base Classes. Polymorphism: Virtual Functions, Virtual Attribute and Inheritance, Virtual Functions and Hierarchy, Pure Virtual Functions, Early vs. Late Binding, Run-Time Type ID and Casting Operators: RTTI, Casting Operators, Dynamic Cast.
Unit 4- Templates and Exception Handling:
Templates: Generic Functions, Applying Generic Functions, Generic Classes, The type name and export Keywords, Power of Templates, Exception Handling: Fundamentals, Handling Derived Class Exceptions, Exception Handling Options, Understanding terminate() and unexpected(), uncaught_exception () Function, exception and bad_exception Classes, Applying Exception Handling.
Unit 5- I/O System Basics:
Streams and Formatted 1/O. File I/O: File Classes, File Operations. Namespaces: Namespaces, std Namespace. Standard Template Library: Overview, Container Classes, General Theory of Operation, Lists, string Class, Final Thoughts on STL.
Course Content
Unit 1- Object Oriented Programming Concepts:
-
Introduction of oop’s Concepts
-
Classes and Objects
-
Methods and Messages
-
Abstraction and Encapsulation
-
Inheritance
-
Abstract Classes
-
Polymorphism
-
Introduction to C++:
-
Classes and Objects
-
Structures and Classes
-
Unions and Classes
-
Friend Functions
-
Friend Classes
-
Inline Functions
-
Static Class Members
-
Scope Resolution Operator
-
Nested Classes
-
Local Classes
-
Passing Objects to Functions
-
Returning objects
-
Object assignment
-
Arrays
-
Pointers
-
References
-
Dynamic Allocation Operators :
-
Arrays of Objects
-
Pointers to Objects
-
Type Checking
-
Pointer
-
Pointers to Derived Types
-
Pointers to Class Members
-
References
-
Dynamic Allocation Operators
Unit 2- Function Overloading and Constructors:
Unit 3- Inheritance and Polymorphism: Inheritance:
Unit 4- Templates and Exception Handling:
Unit 5- I/O System Basics:
No Review Yet